This code...
...sometimes produces error:
I wasn't able to find any dependencies in error appearing, it shows at random time, i can run sequence() at least 4 times before error appears, but sometimes it appears immediately after running sequence().
I've tried to rewrite code many times and i've noticed that problem is in arrays. Something works wrong in that code, but i can't understand what exactly. Can you help me?
Question number two: can i store array in some sort of variable, to address it in the future, like this:
?
Code:
variable
begin
FrameNumber;
FramesQuantity;
end
#define display_frame(param1, param2, param3, param4, param5, param6) DisplayGFX(param5, param1, param2, param3, param4); \
ShowWin; \
wait(param6)
#define sequence(param1) FramesQuantity := len_array(string_split(param1, " % ")); \
FrameNumber := 0; \
while FramesQuantity > FrameNumber do \
begin \
display_frame(166, 35, 308, 308, get_array(string_split(param1, " % "), FrameNumber), Delay); \
FrameNumber := FrameNumber + 1; \
end
Code:
sequence("pcx\\valve_30.pcx % pcx\\valve_60.pcx % pcx\\valve_90.pcx")
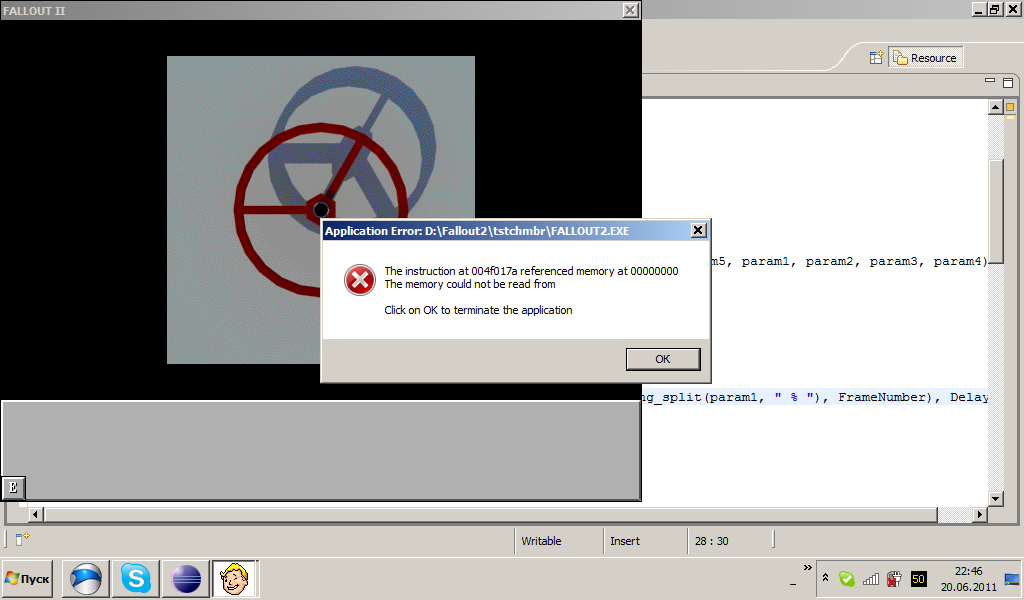
I wasn't able to find any dependencies in error appearing, it shows at random time, i can run sequence() at least 4 times before error appears, but sometimes it appears immediately after running sequence().
I've tried to rewrite code many times and i've noticed that problem is in arrays. Something works wrong in that code, but i can't understand what exactly. Can you help me?
Question number two: can i store array in some sort of variable, to address it in the future, like this:
Code:
TempArray := string_split("element_1 % element_2", " % ");
get_array(TempArray, 0);